Time模块
- time模块基于Unix Timestamp。
- datetime模块基于time进行了封装,提供更多函数。
这两个模块都处理事件,但是对于时间的封装却不同。
- time模块提供struct_time类, 即(time tuple, p_tuple, 时间元组)
- datetime模块常用datetime和timedelta类,也提供了date、time类
python中时间分为
类型 |
所属 |
时间戳 |
time模块,datetime模块 |
时间元组struct_time |
time模块 |
datetime对象 |
datetime模块(又包含date对象和time对象) |
timedelta对象 |
datetime模块 |
time模块中时间表现格式
time模块中时间表现的格式主要有三种:
timestamp时间戳
,时间戳表示的是从1970年1月1日00:00:00开始按秒计算的偏移量
struct_time时间元组
,共有九个元素组。
format time 格式化时间
,已格式化的结构使时间更具可读性。包括自定义格式和固定格式。
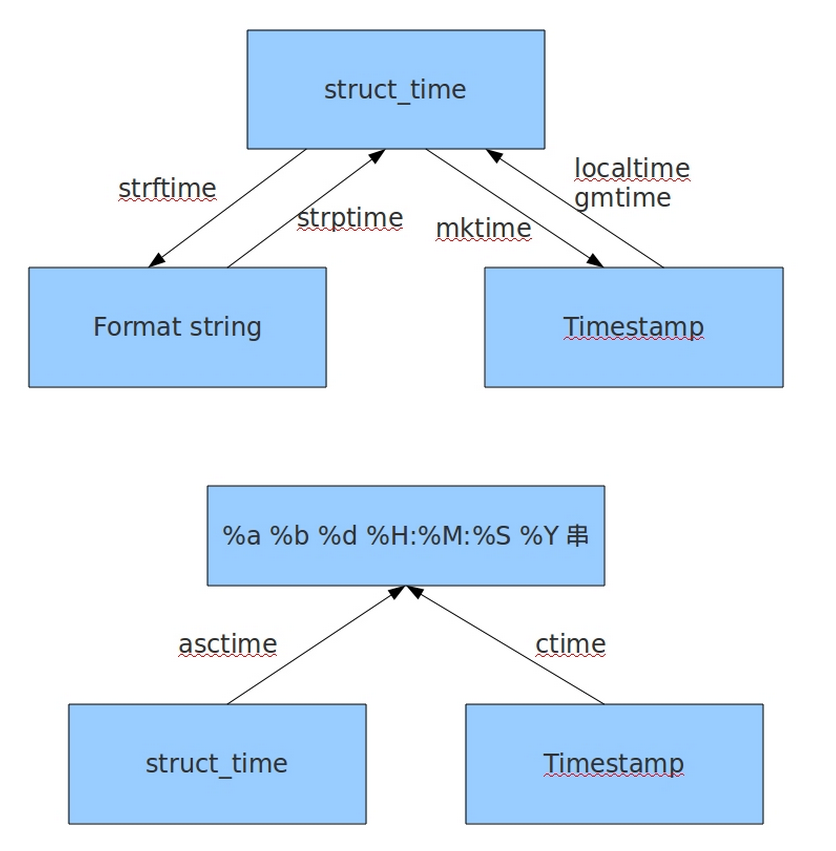
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| import time
p_tuple = time.localtime() print(p_tuple)
print(time.time())
print(time.mktime(p_tuple))
print(time.asctime(p_tuple))
print(time.ctime(time.time()))
print(time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()))
s = '2016-08-25 16:30:58' print(time.strptime(s, "%Y-%m-%d %H:%M:%S"))
|
datetime模块
datetime模块中包含类
类名 |
功能说明 |
date |
日期对象,常用的属性有year, month, day |
time |
时间对象 |
datetime |
日期时间对象,常用的属性有hour, minute, second, microsecond |
timedelta |
时间间隔,即两个时间点之间的长度 |
tzinfo |
时区信息对象 |
datetime_CAPI |
日期时间对象C语言接口 |
date对象
date对象由year年份、month月份及day日期三部分构成:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import datetime
x = datetime.date.today()
a = datetime.date(2017,3,1)
print(x) print(x.year) print(x.month) print(x.day)
print(x.strftime('%Y-%m-%d'))
print(x.timetuple())
|
注意datetime的replace函数,可以直接替换日期.
1 2 3 4 5 6 7
| import datetime
day1 = datetime.datetime.now() day2 = day1.replace(year=2018,day=12)
print(day1) print(day2)
|
time对象
time类由hour小时、minute分钟、second秒、microsecond毫秒和tzinfo五部分组成
1
| time([hour[, minute[, second[, microsecond[, tzinfo]]]]])
|
1 2 3 4 5 6 7 8 9 10
| import datetime
x = datetime.time(12,20,59,889) print(x) print(x.hour) print(x.minute) print(x.second) print(x.microsecond)
print(x.strftime('%H:%M:%S'))
|
datetime类
datetime类其实是可以看做是date类和time类的合体,其大部分的方法和属性都继承于这二个类
1
| datetime(year, month, day[, hour[, minute[, second[, microsecond[,tzinfo]]]]])
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import datetime
a = datetime.datetime.now()
x = datetime.datetime(2017, 3, 22, 16, 9, 33, 494248) print(x)
print(x.date()) print(x.time())
print(type(x.date())) print(type(x.time()))
a = datetime.datetime.now() x = datetime.datetime.combine(a.date(),a.time()) print(x)
x = datetime.datetime.strptime('2017-3-22 15:25','%Y-%m-%d %H:%M') print(x)
|
timedelta类
timedelta类是用来计算二个datetime对象的差值的。
此类中包含如下属性:
- days : 天数
- microseconds :微秒数(>=0 并且 <1秒)
- seconds :秒数(>=0 并且 <1天)
实操
获取当前日期时间
1 2 3 4 5 6 7 8 9 10 11
| import datetime
now = datetime.datetime.now() today = datetime.date.today()
print(now,today)
print(now.time())
|
获取上个月第一天和最后一天的日期
1 2 3 4 5 6 7 8 9 10 11 12
| import datetime
today = datetime.date.today() print(today)
last_day = datetime.date(today.year,today.month,1) - datetime.timedelta(1) print(last_day)
first_day = datetime.date(last_day.year,last_day.month,1) print(first_day)
|
获取时间差
1 2 3 4 5 6 7 8 9 10 11
| import datetime
x = datetime.datetime(2017, 3, 22, 16, 9, 33, 494248) y = datetime.datetime(2014, 2, 23, 12, 19, 24, 49448)
z = x - y print(type(z)) print(z)
print(z.seconds) print(z.days)
|
计算当前时间向后8个小时的时间
1 2 3 4 5 6
| import datetime
now = datetime.datetime.now() x = datetime.timedelta(hours=8)
print(now + x)
|
计算上周一和周日的日期
1 2 3 4 5 6 7 8 9 10 11 12 13
| import datetime
today = datetime.datetime.today() print(today)
today_weekday = today.isoweekday() last_sunday = today - datetime.timedelta(days=today_weekday) last_monday = last_sunday - datetime.timedelta(days=6)
print(today_weekday) print(last_sunday) print(last_monday)
|
计算指定日期当月最后一天的日期和本月天数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import datetime
x = datetime.date(2017,12,20) print(x)
if x.month == 12: last_day = datetime.date(x.year+1,1,1) - datetime.timedelta(days=1) else: last_day = datetime.date(x.year,x.month+1,1) - datetime.timedelta(days=1)
print(last_day)
day_num = last_day.day print(day_num)
|
计算指定日期下个月当天的日期
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| import datetime
date = datetime.date(2017,1,30)
def eomonth(day): '''获取本月最后一天的日期''' if day.month == 12: last_day = datetime.date(day.year+1,1,1) - datetime.timedelta(days=1) else: last_day = datetime.date(day.year,day.month+1,1) - datetime.timedelta(days=1) return last_day
def edate(date_object): '''计算指定日期下个月当天的日期''' if date_object.month == 12: next_month_date = datetime.date(date_object.year+1, 1,date_object.day) else: next_month_first_day = datetime.date(date_object.year,date_object.month+1,1) if date_object.day > eomonth(next_month_first_day).day: next_month_date = datetime.date(date_object.year,date_object.month+1,eomonth(next_month_first_day).day) else: next_month_date = datetime.date(date_object.year, date_object.month+1, date_object.day) return next_month_date
print(edate(date))
|
获得本周一至今天的时间段并获得上周对应同一时间段
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import datetime
today = datetime.datetime.now()
this_monday = today - datetime.timedelta(days=today.isoweekday()-1) this_monday = datetime.datetime(this_monday.year,this_monday.month,this_monday.day)
last_monday = this_monday - datetime.timedelta(days=7) last_weekday = today - datetime.timedelta(7)
day_diff = today - this_monday
print(last_monday) print(last_weekday) print(day_diff)
|
calendar模块
- calendar.Calendar(firstweekday=0)
该类提供了许多生成器,如星期的生成器,某月日历生成器
- calendar.TextCalendar(firstweekday=0)
该类提供了按月、按年生成日历字符串的方法。
- calendar.HTMLCalendar(firstweekday=0)
类似TextCalendar,不过生成的是HTML格式日历
函数及描述
calendar.calendar(year, w=2, l=1, c=6, m=3)
返回一个多行字符串格式的year年年历。
calendar.firstweekday()
返回当前每周起始日期的设置。默认返回0,即星期一。
calendar.isleap(year)
闰年返回True,否则False。
calendar.leapdays(y1, y2)
返回y1到y2之间的闰年总数,包含y1,不包含y2。
calendar.month(year, month, w=2, l=1)
返回一个多行字符串格式的year年month月日历。
calendar.monthcalendar(year,month)
返回一个整数的单层嵌套列表。每个子列表装载一个星期。该月之外的日期都为0,该月之内的日期设为该日的日期,从1开始。
calendar.monthrange(year, month)
返回两个整数组成的元组,第一个是该月的第一天是星期几,第二个是该月的天数。(calendar.monthrange(year, month):
此处计算星期几是按照星期一为0计算。
calendar.prcal(year, w=2, l=1, c=6)
等于print calendar.calendar(year)
calendar.prmonth(year, month)
同上。
calendar.setfirstweekday(weekday)
设置每周起始日期码。0(星期一)到6(星期日)
calendar.timegm(tupletime)
和time.gmtime相反,接收一个时间元组,返回该时刻的时间戳(计算机元年之后的秒数)
calendar.weekday(year, month, day)
返回给定日期的星期码,0(星期一)到6(星期日)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import calendar
print(calendar.month(2018, 10))
print(calendar.calendar(2016))
print(calendar.isleap(2012))
print(calendar.leapdays(y1=2007,y2=2013))
print(calendar.monthcalendar(2019, 8))
print(calendar.monthrange(2019,8))
print(calendar.weekday(year=2019, month=8, day=10))
|